Here you’ll learn how to play a short sound clip by using AudioToolbox.framework in Swift language.
We’ve setup an XCode demo project that looks like this:
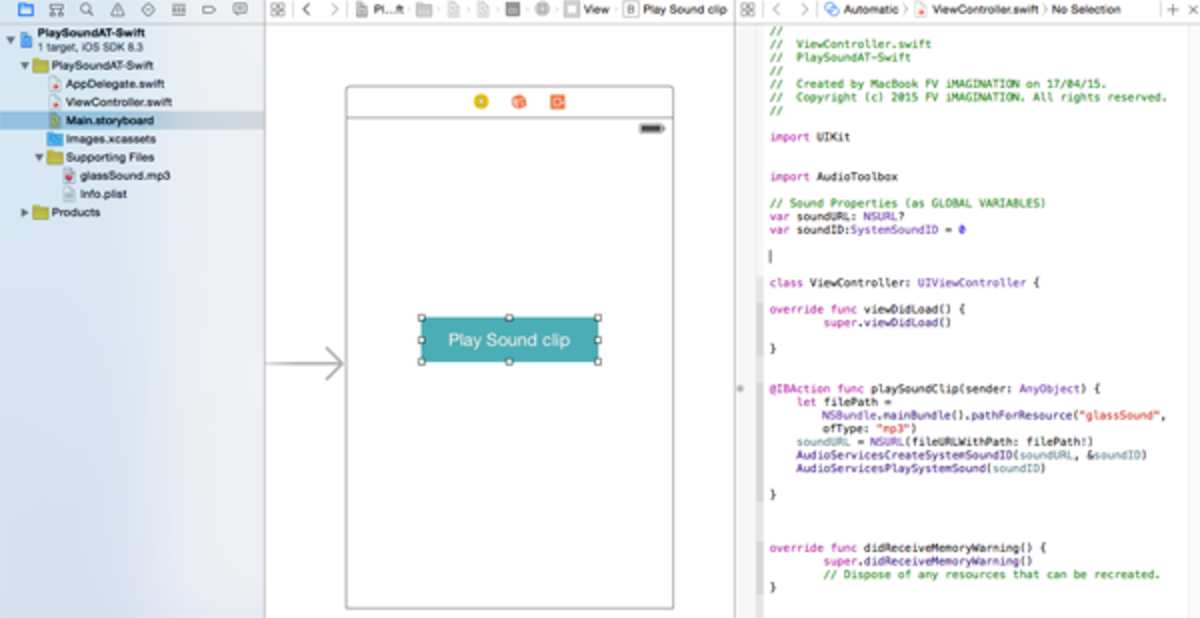
Demo XCode project
Let’s start by importing AudioToolbox into our ViewController.swift file. The great news about the latest version of XCode is that you can import an Apple framework without linking it into Libraries in General tab. All you have to do is to import your needed framework with its real name:
1
| import AudioToolbox |
Awesome, right?
This doesn't mean that if you add this framework to Linked Binary and Libraries in the General tab in XCode that app will not work, you are free to do that if you feel more comfortable. For example, if you want to implement some ad network different than iAd you must link AudioToolbox in that way to your project since their library will need such service.
Ok, we have declared an IBAction of the Play Sound Clip button by ctrl+drag from the button to the .swift file, inside the ViewController class, and before adding the code to play our sound clip, you need to drag a .wav or .mp3 sound file into Supporting Files folder in XCode. The sound file in our demo project is called “glassSound” and it’s an .mp3.
One more thing, we must create a couple of global variables for soundURL and SystemSoundID, you can put them right below the imports:
1
2
| var soundURL: NSURL? var soundID:SystemSoundID = 0 |
And here’s the code to make our Play button play our sound clip:
1
2
3
4
5
6
| @IBAction func playSoundClip(sender: AnyObject) { let filePath = NSBundle.mainBundle().pathForResource( "glassSound" , ofType: "mp3" ) soundURL = NSURL(fileURLWithPath: filePath!) AudioServicesCreateSystemSoundID(soundURL, &soundID) AudioServicesPlaySystemSound(soundID) } |
Let’s check what happens here line by line:
- This is our IBAction for the play button
- Here we get the path of our sound file, which is stored into the device default document directory and it’s called “glassSound” and its type is “mp3″
- Here we get the URL of our sound file as an NSURL with the global variable declared before
- Create a SystemSoundID, needed to play the sound clip later
- Finally play the sound calling Audio Services, which is a property of AudioToolbox framework.
Pretty easy, right? Run your project and tap play to check it out. There’s nothing else to do with AudioToolbox in this tutorial, it has no delegates and it’s mostly used to play short sound clips. You can add some new buttons and repeat the steps mentioned above to play multiple sounds, just assign a different clip to each button.
If you want to play long music files you need to use AVAudioPlayer, which is part of AVFoundation framework. You can find a tutorial about that property on our profile.
No comments:
Post a Comment